« Previous 1 2 3 Next »
Explore automation-as-code with Ansible
Automated
The everything-as-code trend is popular in IT today. The complete hardware and software configuration is stored in text files, and an automation tool rolls everything out. Fortunately, arcane file formats (e.g., sendmail.cf
) are pretty much a thing of the past; instead, you can expect easily readable formats such as YAML and perhaps JSON. The information in these files can usually be converted into one of the other formats without loss.
YAML is the preferred format of the Ansible automation tool, which is used here for as-code automation. Thanks to its modular structure, Ansible can control pretty much everything in the data center and the cloud, including rolling itself out from its command line. This scenario might sound strange, but in the automation-as-code example, it makes sense to roll out a complete AWX setup in this way on Kubernetes and configure the AWX server with all the required settings.
The code here can act as a skeleton, or a template if you prefer, for your own more extensive as-code scenarios, as well as for cases in which you roll out and configure other applications. Because a huge amount of code is involved, I can only describe fragments of it in detail here. The complete code is available on GitHub [1]. Incidentally, it works with both the free AWX and, with a few minor changes, the commercial Ansible automation controller implementation.
Two Approaches
The first approach to automating the AWX rollout involves setting up an empty AWX server, configuring it in the web user interface (UI), and then exporting the complete configuration as a JSON or YAML file. You can then upload the results to a new, empty AWX server. The approach is mainly used if you want to clone a setup or migrate to a new platform or a new release (e.g., Ansible Tower to Ansible controller).
However, this approach
...Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
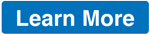