« Previous 1 2 3
Angular 2 client-side TypeScript framework
Second Brew
After no fewer than six years of development, the second major release of the Angular client-side JavaScript framework [1] was released in mid-September 2016. The good news: Angular 2 also relies on data bindings to place application data in a browser HTML document using directives (i.e., specifically defined HTML tags). The bad news: Angular 2 is not downwardly compatible, although not so much because of the codebase rewrite under TypeScript [2], but rather because of design decisions made by the Angular team.
In this article, I refer to a sample application (Figure 1) to describe how developers can program apps in Angular 2 with TypeScript. The app is simple: Clicking on Add
transfers the two measured values for temperature and pressure from the fields in the form to the table at the bottom of the output window. At the same time, the app calculates the average of the measured values in the line below the Weather Station
label.
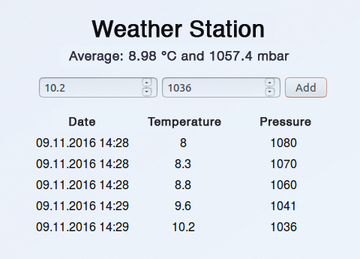
Quick
...Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
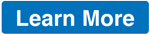