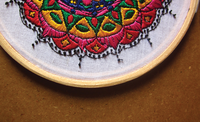
Photo by Santoshi Guruju on Unsplash
Program GUIs in Go with Fyne
Fyne Work
Creating a graphical user interface (GUI) with the Fyne framework [1] requires some preparation, including installing the GCC compiler and a graphics driver [2]. You will also need at least Go v1.12. The command
go get fyne.io/fyne/v2
downloads and sets up Fyne v2.
To get a first impression of the different Fyne widgets, you can take a look at a demo app [3] and its available controls. To download and launch this Fyne demo, enter
go get fyne.io/fyne/v2/cmd/fyne_demo fyne_demo
in the terminal.
Basic Framework
A few lines of code are all it takes to create a spartan window in Fyne (Listing 1). The import
statement brings in the required packages. To create an executable program, line 9 defines the main()
function as the entry point. The app.New()
method creates a new Fyne instance, and the a.NewWindow("<Title>")
method specifies a title for the header of the newly created program window.
Listing 1
Basic Fyne Framework
01 package main 02 03 import ( 04 "fyne.io/fyne/v2" 05 "fyne.io/fyne/v2/app" 06 "fyne.io/fyne/v2/widget" 07 ) 08 09 func main() { 10 a := app.New() 11 w := a.NewWindow("<Title>") 12 w.Resize(fyne.NewSize(200, 200)) 13 w.SetContent(widget.NewLabel("Hello World!")) 14 w.CenterOnScreen() 15 w.ShowAndRun() 16 }
As you might expect from the last four lines, the program makes the window appear
...Buy this article as PDF
(incl. VAT)